Testing Mailer in Go
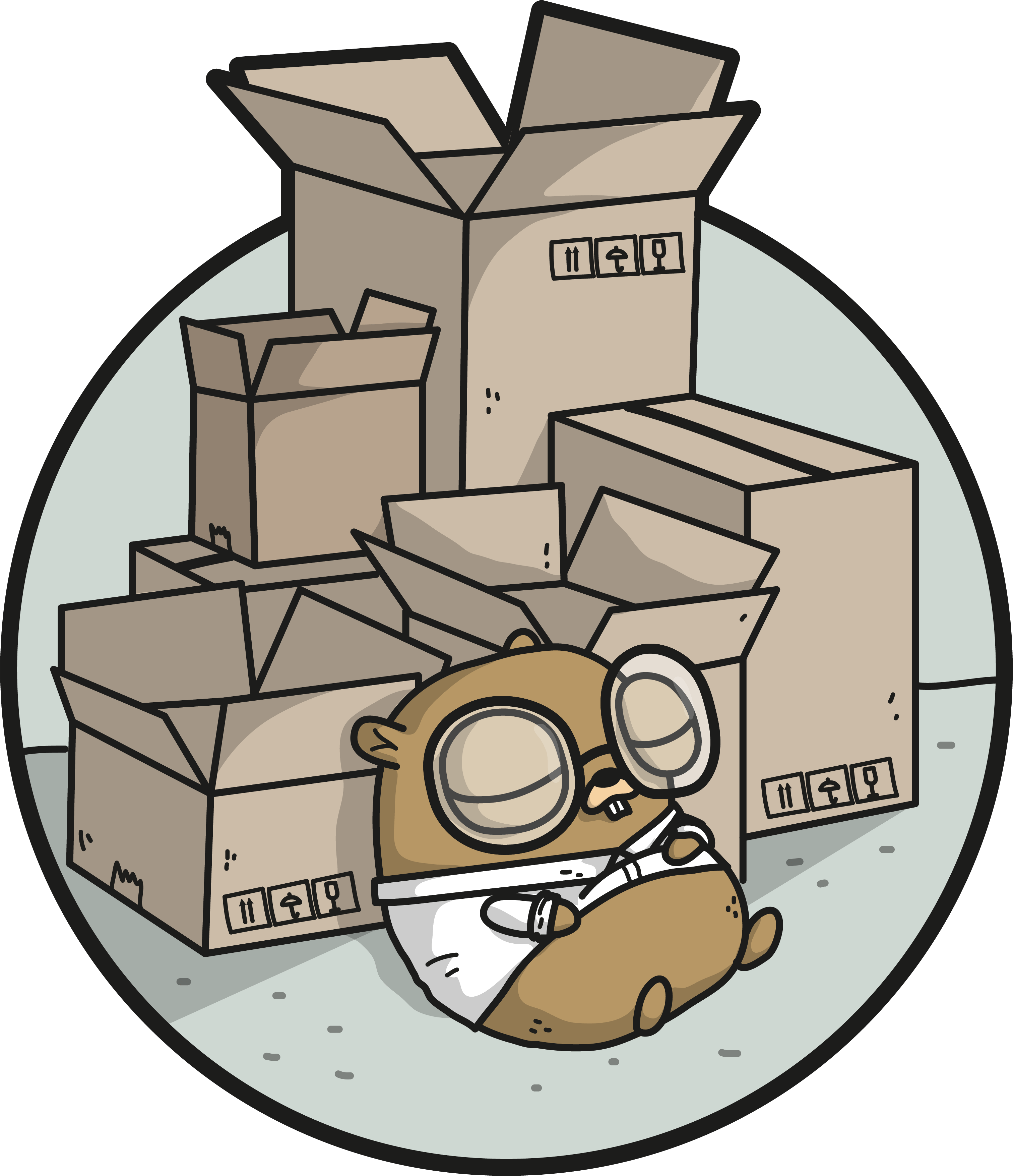
Forewords
I needed a way to test mail confirmation. When you are dealing with deb authentication you would like to be sure that this email exist. And usually I use is_email_confirmed
column next to user credentials in the database table. Once user hits siging endpoint as last step I send an email with confirmation token.
I was looking on few popular tools across the Internet:
What I found
My requirements were: run in docker, easy to config, basic functionality to render email. All things above quite heavy apps with complex configuration. After that I found found nice and lightweight way how to test it locally.
-> https://github.com/mailhog/MailHog
Setup
I did the small package in my project and now I can use it around my services to send emails and catch them in mailhog!
About golang smtp you can checkout here
I would recommend setting this up via env, check my post about it.
Not sure if it will be useful to anyone, but i decided to share it.
Cover from github.com/ashleymcnamara/gophers.